Update 6. June 2018: Starting from version 1.1.0, SneakyThrow doesn't wrap the exception into a RuntimeException.
Are you tired of writing "catch-pseudo-code" for Java's Checked Exceptions? And let’s not think about that annoying additional unit test.
Help is on the way.
Let me introduce you to SneakyThrow. Cut back the amount of code you need to write to catch Checked Exceptions thanks to this Java library.
It uses only “legal stuff” — no magic like bytecode manipulation. I am looking at you Project Lombok 😉
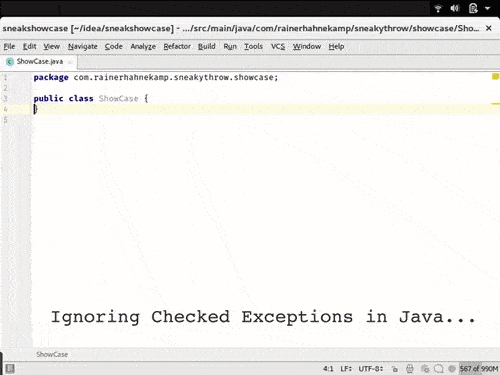
How can I get it?
Simply copy & paste the library as a dependency into your pom.xml or similar dependency manager of choice:
<dependency> <groupId>com.rainerhahnekamp</groupId> <artifactId>sneakythrow</artifactId> <version>1.1.0</version> </dependency>
How can I use it?
Without SneakyThrow, code with Checked Exceptions looks like that:
URL url; try { url = new URL("https://www.hahnekamp.com"); } catch (MalformedURLException mue) { throw new RuntimeException(mue); }
Thanks to SneakyThrow, your code looks like this:
URL url = sneak(() -> new URL("https://www.hahnekamp.com"));
Great! Does it also work with Stream and lambdas?
Sure thing. Once again the code without SneakyThrow:
private URL createURL(String url) throws MalformedURLException { return new URL(url); } Stream .of("https://www.hahnekamp.com", "https://www.austria.info") .map(url -> { try { return this.createURL(url); } catch (MalformedURLException mue) { throw new RuntimeException(mue); } }) .collect(Collectors.toList());
And now again much less code with SneakyThrow:
private URL createURL(String url) throws MalformedURLException { return new URL(url); } Stream .of("https://www.hahnekamp.com", "https://www.austria.info") .map(sneaked(this::createURL)) .collect(Collectors.toList());
Please note the difference in sneak and sneaked.
Where can I get more info?
The article "Ignore Exceptions in Java" describes the rationale and the basic approach behind SneakyThrow in more detail.
SneakyThrow is OpenSource and available on GitHub.
Try it out and let me hear how it is going. Feedback of any kind is welcome.